Angular 2 and OnSen UI based hybrid News feed app
Angular 2 and OnSen UI based hybrid News feed app, the news are fetched from newsapi.org (over 50 sources).
The overall native feel of Onsen UI on iOS is amazing but I guess they will have to work hard to match what Ionic has to offer. The Source is available on my github repository for you to clone and enjoy.
Source Code: https://github.com/gognamunish/ng2-onsen_ui-newsfeed
Screenshots
Source Code: https://github.com/gognamunish/ng2-onsen_ui-newsfeed
Screenshots
![]() | ![]() |
Ionic + FireBase based Expenditure tracker app
This time I tried my hands on the Ionic framework, find the details below:
https://github.com/gognamunish/ionic-expenditure-tracker Thanks, Happy New Year !!!
https://github.com/gognamunish/ionic-expenditure-tracker Thanks, Happy New Year !!!
ng2 based Order Entry System for learning purpose
This weekend I could finally manage time to explore Angular 2 framework thanks to Sachin Shah for coming up with idea to explore the hidden gems in modern web technologies.
Have a look at READ ME page on my Github page below:
Feel free to clone and play with it.
This project uses a number of open source projects to work properly:
- [AngularJS] - HTML enhanced for web apps!
- [Spring Boot] - Awesome boot framework
- [Spring Data] - We are using JPA repository
- [Spring Security] - Just for basic authentication
- [HSQL] - Embedded database.
- [Ng Material] - Material Design components for Angular 2
- [Rest Assured] - Testing and validating REST services
Have a look at READ ME page on my Github page below:
Feel free to clone and play with it.
Acceptance Tests using Cucumber
Most of the Systems are buggy due to lack of Acceptance tests, so in today's post I will try to walk you through a simple Cucumber based acceptance test for the following very common JIRA User story.
Step 1 : Describe behavior of the Test in plain English (roles2functions.feature)
In this .feature file We’ve translated one of the acceptance criteria we were given in the JIRA task into a Cucumber scenario that we can ask the computer to run over and over again. The keywords Feature, Scenario, When, and Then are the structure, and everything else is documentation.
Step 2 : Implementing Our Step Definitions
Let's run our Fixtures using Cucumber runner as shown below:
1 Scenarios (1 passed)
2 Steps (2 passed)
0m0.310s
In case if the test is failing the logs will have enough information about the Assertion that was made.
Summary from this post
Software teams work best when the developers and business stakeholders are communicating clearly with one another. A great way to do that is to collaboratively specify the work that’s about to be done using automated acceptance tests (and the good thing about Cucumber is that they can be imported directly from User stories in JIRA).
In the next article I would evaluate Fitnesse for the same feature.
As a RM User of the System I want to make sure that entitlements are properly set So that I am able to Place Orders in the new Order Entry System.Each test case in Cucumber is called a scenario, and scenarios are grouped into features. Each scenario contains several steps. The good thing about Cucumber is that your JIRA story can be taken as is to form the basis of acceptance test so that Business and Developers can be in talking terms with each other. I am skipping the Maven wiring for the project and jumping directly into the feature that we want to implement in this post.
Step 1 : Describe behavior of the Test in plain English (roles2functions.feature)
Feature: User Entitlement Scenario: User of the Bank in certain role would like to access certain functionality of existing System. When The logged In User "U12345" has standard "RM" role Then "Place Orders" functionality of "OES" application should be available to him/herThere is a scope for enriching this feature description but for the sake of the goal of this post (introducing you to Cucumber), we are good to go.
In this .feature file We’ve translated one of the acceptance criteria we were given in the JIRA task into a Cucumber scenario that we can ask the computer to run over and over again. The keywords Feature, Scenario, When, and Then are the structure, and everything else is documentation.
Step 2 : Implementing Our Step Definitions
package com.gognamunish; import static org.hamcrest.CoreMatchers.is; import static org.hamcrest.MatcherAssert.assertThat; import static org.hamcrest.Matchers.equalTo; import java.util.List; import org.junit.Assert; import cucumber.api.java.en.Then; import cucumber.api.java.en.When; /** * A very basic implementation for the Acceptance criteria. * @author Munish Gogna */ public class EntitlementFixtures { String role; EntitlementService entitlementService = new EntitlementService(); @When("^The logged In User \"(.*?)\" has standard \"(.*?)\" role$") public void the_logged_In_User_has_role(String userId, String role) throws Throwable { Assert.assertNotNull(role); Assert.assertNotNull(userId); this.role = role; List<String> userRoles = entitlementService.getUserRoles(userId); assertThat(true, equalTo(userRoles.contains(role))); } @Then("^\"(.*?)\" functionality of \"(.*?)\" application should be available to him/her$") public void functionality_of_application_should_be_available_to( String function, String application) throws Throwable { Assert.assertNotNull(function); Assert.assertNotNull(application); List<String> functions = entitlementService .getFunctionsForRoleAndApplication(role, application); assertThat(true, equalTo(functions.contains(function))); } }
Let's run our Fixtures using Cucumber runner as shown below:
package com.gognamunish; import org.junit.runner.RunWith; import cucumber.api.junit.Cucumber; @RunWith(Cucumber.class) public class RunEntitlementTest { // intentional }Well well well - all tests have passed :)
1 Scenarios (1 passed)
2 Steps (2 passed)
0m0.310s
In case if the test is failing the logs will have enough information about the Assertion that was made.
Summary from this post
Software teams work best when the developers and business stakeholders are communicating clearly with one another. A great way to do that is to collaboratively specify the work that’s about to be done using automated acceptance tests (and the good thing about Cucumber is that they can be imported directly from User stories in JIRA).
In the next article I would evaluate Fitnesse for the same feature.
SphinxQL over JDBC
This is an extension of my earlier blog post about performing free text search with Sphinx (v2.1.6+).
This time we are going to access the index via JDBC as opposed to Sphinx API and perform some basic queries to fetch indexed data.
Add following entries to searchd section inside sphinx.conf
Once done start the sphinx daemon (searchd) so that the search engine can be accessed from external applications on port 9306. Next just get the JDBC connection (no need to specify database details and credentials) as shown below and you are ready to rock.
Note:
Add following entries to searchd section inside sphinx.conf
listen=9306:mysql41 mysql_version_string=5.0.37
Once done start the sphinx daemon (searchd) so that the search engine can be accessed from external applications on port 9306. Next just get the JDBC connection (no need to specify database details and credentials) as shown below and you are ready to rock.
Class.forName("com.mysql.jdbc.Driver"); con = DriverManager .getConnection("jdbc:mysql://localhost:9306"); statement = con.createStatement(); // perform wild search on last names resultSet = statement .executeQuery("select * from addressBookIndex where MATCH ('gogna | sharma')");
Note:
- 1. The attributes specified in the sphinx.conf file (e.g. sql_attr_string, sql_attr_uint etc) can not be used with MATCH though they can be part of WHERE clause.
- 2. Given that now String attributes are supported in order to avoid making a database call define key columns as attributes as well as fields in sql_query.
- 3. Results from Delta Indexes can be handled using union.
- 4. Explore RT indexes for real time update scenarios.
Apache Velocity Template to generate CSV (or any other format) file
This post is simple one to generate CSV file from some Source using Velocity Template and to verify the new design for the Blog (Metro Blue). The code (just a running stuff and nothing fancy) provided is simple and doesn't need any explanation and is dedicated to a dear friend Sachin Shah.
Java Class
POM Dependency
Java Class
package com.gognamunish.test; import org.apache.velocity.app.Velocity; import org.apache.velocity.Template; import org.apache.velocity.VelocityContext; import java.io.File; import java.io.FileWriter; import java.io.StringWriter; import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.ArrayList; import java.util.Date; import java.util.HashMap; import java.util.List; import java.util.Map; public class CSVFeedGeneration { static DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss"); static DataFeed dataFeed; static { // prepare some static data dataFeed = new DataFeed(); List<Map<String, Object>> dataList = new ArrayList<Map<String, Object>>(); Map<String, Object> data = new HashMap<String, Object>(); data.put("TransactionId", 1); data.put("Amount", new Integer(2312)); data.put("Currency", "SGD"); data.put("ExecutionDate", dateFormat.format(new Date())); dataList.add(data); data = new HashMap<String, Object>(); data.put("TransactionId", 2); data.put("Amount", new Integer(1212)); data.put("Currency", "INR"); data.put("ExecutionDate", dateFormat.format(new Date())); data.put("Status", "Active"); dataList.add(data); dataFeed.getFeedData().add(dataList); } public static void main(String[] args) throws Exception { VelocityContext context = new VelocityContext(); FileWriter writer = new FileWriter(new File("feed.csv")); // inject the objects to be used in template context.put("feed", dataFeed); context.put("DELIMETER", ","); context.put("NEWLINE", "\n"); Velocity.init(); Template template = Velocity.getTemplate("csvfeed.vm"); template.merge(context, writer); writer.flush(); writer.close(); } }Template File (csvfeed.vm)
TransactionId${DELIMETER}Amount${DELIMETER}ExecutionDate ${DELIMETER}Currency${DELIMETER}Status$NEWLINE #foreach( $rowSet in $feed.feedData) #foreach( $row in $rowSet ) $!row.get("TransactionId")$DELIMETER$!row.get("Amount") $DELIMETER$!row.get("ExecutionDate")$DELIMETER $!row.get("Currency")$DELIMETER$!row.get("Status")$NEWLINE #end #endOutput File (feed.csv)
TransactionId,Amount,ExecutionDate,Currency,Status 1,2312,2013-08-18T14:29:35,SGD, 2,1212,2013-08-18T14:29:35,INR,ActiveThe main advantage of this approach is that you can change the data format any time without changing or touching any Java code.
POM Dependency
<dependency> <groupId>org.apache.velocity</groupId> <artifactId>velocity</artifactId> <version>1.7</version> </dependency>In case you are interested in exploring more, reach out here http://velocity.apache.org/engine/devel/index.html
Configuring IBM MQ in JBoss
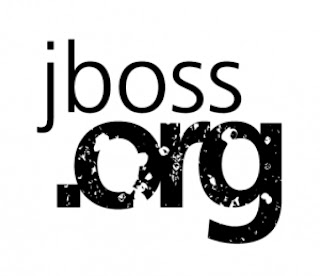
In today's post I will explain how to install the WebSphere MQ resource adapter on JBoss.
Installing WebSphere MQ resource adapter:
To install WebSphere MQ resource adapter, copy the file wmq.jmsra.rar (from installation directory) to the server's deploy directory, for example <install path>/server/default/deploy The resource adapter will be automatically picked up by the server.
Installing the WebSphere MQ Extended Transactional Client:
The WebSphere MQ Extended transactional client lets you use XA distributed transactions with CLIENT mode connections to a WebSphere MQ queue manager. To install it on JBoss, copy the file com.ibm.mqetclient.jar ( from installation directory ) to the server lib directory, for example <install path>/server/default/lib.
Configuring the resource adapter for your application:
The WebSphere MQ resource adapter lets you define a number of global properties. Resource definitions for outbound JCA flows are defined in a JBoss -ds.xml file named wmq.jmsra-ds.xml. The outline form of this file:
As can be seen above JCA connection factories are defined in the <tx-connection-factory> element and JMS queues and topics are defined as JCA administered object mbeans.
A complete example for our sample application defining two queues - inbound and outbound is given below:
The place holder properties are defined in the JBoss start script as:
-Dchannel=MQTEST -DhostName=localhost -DqueueManager=TST_MGR
Configuring inbound message delivery:
Message delivery to a Message-Driven Enterprise JavaBean (MDB) is configured using a JBoss-specific file named jboss.xml, located in the MDB .ear file. The contents of this file will vary, but will be of the form:
jboss.xml:
The DestinationType and Name properties must be specified. Other properties are optional, and if omitted, take on their default values.
A simple MDB definition for the inbound message is shown below:
A plain java class to send message to the output queue is shown below.
That's all.
Installing WebSphere MQ resource adapter:
To install WebSphere MQ resource adapter, copy the file wmq.jmsra.rar (from installation directory) to the server's deploy directory, for example <install path>/server/default/deploy The resource adapter will be automatically picked up by the server.
Installing the WebSphere MQ Extended Transactional Client:
The WebSphere MQ Extended transactional client lets you use XA distributed transactions with CLIENT mode connections to a WebSphere MQ queue manager. To install it on JBoss, copy the file com.ibm.mqetclient.jar ( from installation directory ) to the server lib directory, for example <install path>/server/default/lib.
Configuring the resource adapter for your application:
The WebSphere MQ resource adapter lets you define a number of global properties. Resource definitions for outbound JCA flows are defined in a JBoss -ds.xml file named wmq.jmsra-ds.xml. The outline form of this file:
As can be seen above JCA connection factories are defined in the <tx-connection-factory> element and JMS queues and topics are defined as JCA administered object mbeans.
A complete example for our sample application defining two queues - inbound and outbound is given below:
jms/MyAppConnectionFactory wmq.jmsra.rar true javax.jms.QueueConnectionFactory 8 36 ${channel} ${hostName} 1414 ${queueManager} CLIENT munish JmsXARealm jms/IncomingQueue jboss.jca:name='wmq.jmsra.rar',service=RARDeployment javax.jms.Queue baseQueueManagerName=${queueManager} baseQueueName=MY.APP.INBOUND.QUEUE jms/OutgoingQueue jboss.jca:name='wmq.jmsra.rar',service=RARDeployment javax.jms.Queue baseQueueManagerName=${queueManager} baseQueueName=MY.APP.OUTBOUND.QUEUE
The place holder properties are defined in the JBoss start script as:
-Dchannel=MQTEST -DhostName=localhost -DqueueManager=TST_MGR
Configuring inbound message delivery:
Message delivery to a Message-Driven Enterprise JavaBean (MDB) is configured using a JBoss-specific file named jboss.xml, located in the MDB .ear file. The contents of this file will vary, but will be of the form:
jboss.xml:
MyMessageBean DestinationType javax.jms.Queue destination MY.APP.INBOUND.QUEUE channel ${channel} hostName ${hostName} port 1414 queueManager ${queueManager} transportType CLIENT username munish wmq.jmsra.rar
The DestinationType and Name properties must be specified. Other properties are optional, and if omitted, take on their default values.
A simple MDB definition for the inbound message is shown below:
/** * This message driven bean is used to collect asynchronously received messages. * * @author Munish Gogna */ @MessageDriven(mappedName = "jms/IncomingQueue") public final class MyMessageBean implements javax.jms.MessageListener { private static final org.apache.log4j.Logger LOGGER = org.apache.log4j.Logger.getLogger(MyMessageBean.class); public void onMessage(final javax.jms.Message message) { LOGGER.info("Received message: " + message); // TODO - do processing with the message here } }
A plain java class to send message to the output queue is shown below.
/** * This class is used to send text messages to a queue. * * @author Munish Gogna */ public final class MessageSender { private static final org.apache.log4j.Logger LOGGER = org.apache.log4j.Logger.getLogger(MessageSender.class); /** * A coarse-grained method, nothing fancy. In actual applications please re factor the code and * handle the exceptions properly. * * @throws Exception */ public void send(final String message) throws Exception { if (message == null) { throw new IllegalArgumentException("Parameter message cannot be null"); } javax.jms.QueueConnection connection = null; javax.jms.Session session = null; try { javax.naming.Context fContext = new javax.naming.InitialContext(); javax.jms.QueueConnectionFactory fConnectionFactory = (javax.jms.QueueConnectionFactory) fContext .lookup("java:jms/MyAppConnectionFactory"); connection = fConnectionFactory.createQueueConnection(); session = connection.createSession(false, javax.jms.Session.AUTO_ACKNOWLEDGE); final javax.naming.Context jndiContext = new javax.naming.InitialContext(); final javax.jms.Destination destination = (javax.jms.Destination) jndiContext.lookup("jms/OutgoingQueue"); final javax.jms.MessageProducer messageProducer = session.createProducer(destination); final javax.jms.TextMessage textMessage = session.createTextMessage(message); messageProducer.send(textMessage); messageProducer.close(); } catch (Exception nex) { throw nex; } finally { if (session != null) { try { session.close(); } catch (JMSException e) { LOGGER.error("Failed to close JMS session", e); } } if (connection != null) { try { connection.close(); } catch (JMSException e) { LOGGER.error("Failed to close JMS connection", e); } } } } }A utilty called RFHUTILC.EXE can be used to connect to remote queues.
That's all.
Saving development time with local EntityManager in JPA
In almost all real world projects (non Spring + We are not considering Arquillian or any similar framework) where you are using JPA (Hibernate implementation), the data source binding happens by JNDI and the services are provided by EJBs, which means to test your entities for any CRUD operation you have to deploy the bundle (ear, ejb or war) into the application server which adds time to your development efforts each time you make any change.
So in this post we will see how we can temporarily inject entity manager into your business class so that you can test all the operations you want to perform on entities without deploying the application or restarting server quite often.
persistence.xml: The configuration for entity managers both inside an application server and in a standalone application reside in a persistence archive. In the next section we focus on standalone configuration.
Let's try one sample example:
Hibernate: select entity0_.id as id0_, entity0_.value as value0_ from Entity entity0_
Before Entities: []
Hibernate: select entity0_.id as id0_0_, entity0_.value as value0_0_ from Entity entity0_ where entity0_.id=?
Hibernate: insert into Entity (value, id) values (?, ?)
Hibernate: select entity0_.id as id0_, entity0_.value as value0_ from Entity entity0_
After Entities: [Id:1, Value:my first value]
persistence.xml: The configuration for entity managers both inside an application server and in a standalone application reside in a persistence archive. In the next section we focus on standalone configuration.
Please Note:org.hibernate.ejb.HibernatePersistence com.gognamunish.test.Entity
- The database dialect we have chosen is MySQL for obvious reasons.
- Persistence unit has name test_unit and the type is RESOURCE_LOCAL and not JTA.
- com.gognamunish.test.Entity is the entity which we want to test later.
- As we are running without a JNDI available datasource, we must specify JDBC connections with Hibernate specific properties
EntityManagerFactory factory ; factory = Persistence.createEntityManagerFactory("test_unit"); EntityManager manager = factory.createEntityManager();That's all you need , after obtaining the reference focus only on your entity and the operation you want to perform on it.
Let's try one sample example:
/* Sample Entity class */ @javax.persistence.Entity @Table(name = "Entity") public class Entity { @Id private Long id; @Column private String value; }
package com.gognamunish.test; import java.util.List; import javax.persistence.EntityManager; import javax.persistence.EntityManagerFactory; import javax.persistence.Persistence; /** * Sample Test - nothing fancy. * * @author Munish Gogna * */ public class LocalEntityManager { static EntityManager manager; static { EntityManagerFactory factory = Persistence .createEntityManagerFactory("test_unit"); manager = factory.createEntityManager(); } public static void main(String[] args) { System.out.println("Before Entities: " + getEntities()); manager.getTransaction().begin(); Entity entity = new Entity(); entity.setId(1l); entity.setValue("my first value"); saveOrUpdate(entity); manager.getTransaction().commit(); System.out.println("After Entities: " + getEntities()); } private static Entity saveOrUpdate(Entity entity) { return manager.merge(entity); } @SuppressWarnings("unchecked") private static List<Entity> getEntities() { return manager.createQuery("from Entity").getResultList(); } }LOGS:
Hibernate: select entity0_.id as id0_, entity0_.value as value0_ from Entity entity0_
Before Entities: []
Hibernate: select entity0_.id as id0_0_, entity0_.value as value0_0_ from Entity entity0_ where entity0_.id=?
Hibernate: insert into Entity (value, id) values (?, ?)
Hibernate: select entity0_.id as id0_, entity0_.value as value0_ from Entity entity0_
After Entities: [Id:1, Value:my first value]
Reverse a String in Java
One of the frequently asked questions in interviews, let's try few ways available in JDK to reverse a string:
import java.util.Stack; public class StringReverse { public static String reverse1(String s) { int length = s.length(); if (length <= 1) return s; String left = s.substring(0, length / 2); String right = s.substring(length / 2, length); return reverse1(right) + reverse1(left); } public static String reverse2(String s) { int length = s.length(); String reverse = ""; for (int i = 0; i < length; i++) reverse = s.charAt(i) + reverse; return reverse; } public static String reverse3(String s) { char[] array = s.toCharArray(); String reverse = ""; for (int i = array.length - 1; i >= 0; i--) reverse += array[i]; return reverse; } public static String reverse4(String s) { return new StringBuffer(s).reverse().toString(); } public static String reverse5(String orig) { char[] s = orig.toCharArray(); int n = s.length - 1; int halfLength = n / 2; for (int i = 0; i <= halfLength; i++) { char temp = s[i]; s[i] = s[n - i]; s[n - i] = temp; } return new String(s); } public static String reverse6(String s) { char[] str = s.toCharArray(); int begin = 0; int end = s.length() - 1; while (begin < end) { str[begin] = (char) (str[begin] ^ str[end]); str[end] = (char) (str[begin] ^ str[end]); str[begin] = (char) (str[end] ^ str[begin]); begin++; end--; } return new String(str); } public static String reverse7(String s) { char[] str = s.toCharArray(); Stack<Character> stack = new Stack<Character>(); for (int i = 0; i < str.length; i++) stack.push(str[i]); String reversed = ""; for (int i = 0; i < str.length; i++) reversed += stack.pop(); return reversed; } }Feel free to suggest other ways if you have.
Merging PDFs while retaining the fill able forms inside them
My previous post was about merging the PDF files into single file using PDFBox, the limitation of that approach was if you had fill able form inside any PDF file it was lost, to over come that issue here is an updated version of the same post using iText library.
The iText jar can be downloaded here
Thanks.
import java.io.FileOutputStream; import java.io.InputStream; import java.io.OutputStream; import java.net.URL; import java.util.ArrayList; import java.util.List; import java.util.Set; import com.lowagie.text.pdf.AcroFields; import com.lowagie.text.pdf.PdfCopyFields; import com.lowagie.text.pdf.PdfReader; /** * Merges the PDF files while retaining the fillable forms within them. It also * renames form field (to avoid conflicts) which can have an impact if form is * submitted to server but for printing purpose should be OK. * * @author Munish Gogna * */ public class PDFMerge { private static int counter = 0; public static void merge(List<InputStream> sourcePDFs, OutputStream targetPDF) throws Exception { if (sourcePDFs != null && targetPDF != null) { PdfCopyFields copy = new PdfCopyFields(targetPDF); for (InputStream stream : sourcePDFs) { PdfReader pdfReader = new PdfReader(stream); renameFields(pdfReader.getAcroFields()); copy.addDocument(pdfReader); stream.close(); } copy.close(); } } private static void renameFields(AcroFields fields) { Set<String> fieldNames = fields.getFields().keySet(); String prepend = String.format("_%d.", counter++); for (String fieldName : fieldNames) { fields.renameField(fieldName, prepend + fieldName); } } public static void main(String[] args) { try { List<InputStream> pdfs = new ArrayList<InputStream>(); pdfs .add(new URL( "http://thewebjockeys.com/TheWebJockeys/Fillable_PDF_Sample_from_TheWebJockeys_vC5.pdf") .openStream()); pdfs .add(new URL( "http://help.adobe.com/en_US/Acrobat/9.0/Samples/interactiveform_enabled.pdf") .openStream()); OutputStream output = new FileOutputStream("merged.pdf"); merge(pdfs, output); } catch (Exception e) { e.printStackTrace(); } } }
The iText jar can be downloaded here
Thanks.